Programming languages is not the easiest to be differentiated and it often comes in a variety of styles, shapes, and expressions. But one common thing many programmers can agree is by classifying the languages by organizing them into a paradigm. And these paradigms come in different functionalities and terms like procedural programming, functional, object-oriented, imperative and so on.
It’s hard to deny that these terms can be quite confusing especially for those who are starting to dipping its toe into the programming world. Hence, here we are to clarify the whole situation and to give you a clearer picture of imperative programming.
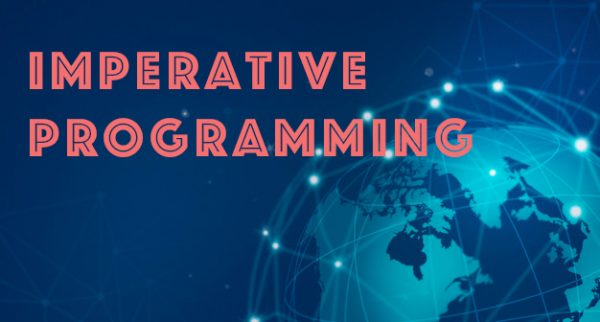
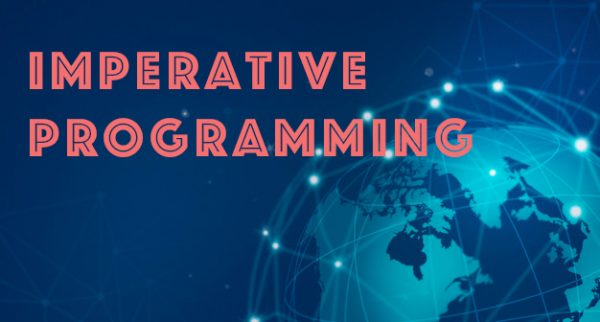
What is Imperative Programming?
Imperative programming is a type of programming paradigm which uses statements that describe a step by step process that changes the state of a program. However, this programming paradigm is the opposite of declarative programming. While declarative programming is elaborated on what a certain program should accomplish. On the other hand, imperative programming clearly explains the program how it should accomplish.
Using imperative programming, it needs a detailed understanding of the functions needed to solve the problem. While a computer program is written in an imperative way of coding compile binary executables that run on all CPU instructions. Low-level programming like machine language programmings such as assembly programming, C programming, and COBOL programming is using an imperative style.
The idea of imperative programming was established way back in the 1950s to make the lives of programmers simpler and easier to read and write code. Code blocks were established where program code was grouped into sections.
These code blocks were also originally called compound statements but later on, in this era of digitalization, it was known as procedures. Using single imperative statements, procedures should be defined allowing programmers to express ideas. Procedural Programming is a type of imperative programming with a step by step process leaning towards a higher-level of programming method a.k.a. declarative programming.
Object-oriented programming, on the other hand, is also a type of imperative programming that focuses on the classes and objects. Well known OOP programming languages are C++, C#, and Java. This type of programming elaborated on the step by step processes on how the problem should be solved.
Reasons to use Imperative Programming
Although, Imperative way of programming has been used a long time ago, and used in the implementation of low-level- machine language programming, let me elaborate to you on the importance of using an imperative way of programming in this era. Here are the characteristics that need to consider.
-
Machine Architecture
Since imperative programming is one of the oldest programming paradigms, programming works by changing the state through assignment statements. This programming paradigm is also used and based on Von Neumann architecture. This implements a step by step task by mutable state. This paradigm has several statements which store the result after execution.
-
Sequential Execution
To illustrate imperative programming, here’s a simple example of how to code imperative programming does in a sequential way of coding.
Console.WriteLine(“How many years do you spend in college?”);
var college = int.Parse(Console.ReadLine());
Console.WriteLine(“You spend {0} years in getting your college degree”, college);
There are only three lines in the example code above. The first line is a static method that asks the user to input the number they spend in college using the syntax Console.WriteLine. The second line is a variable declaration named college which uses an argument int.Parse() to convert characters to an integer. The last line prompts a message to the user informing the number of years spent in college. Naturally imperative way of coding is a step by step process thus, demonstrate the sequential nature of the imperative paradigm.
-
Mutable State
One of the characteristics of imperative programming is that it translates the problem into step by step and execution which leads to simpler implementations. An example code snippet below shows how straightforward implementation of imperative programming.
Console.WriteLine(“How many years do you spend in college?”);
var college = int.Parse(Console.ReadLine());
Console.WriteLine(“You spend {0} years in getting your college degree”, college);
college++;
Console.WriteLine(“Now you’re spending {0} years in college”, college);
This might be a hilarious example but this is the best way to illustrate imperative programming paradigm mutable values. In the first three lines, the code shows a simple straightforward static message using Console.WriteLine and variable declarations.
A variable named college is passed in an argument int.Parse() to convert character input to integer for numbers. In the last line, it prints a message for the user informing the number of years spending in college. Imperative programming is sequential in nature, thus, in our example, it shows an immutability approach on the way of coding. This makes code cleaner and easier to understand.
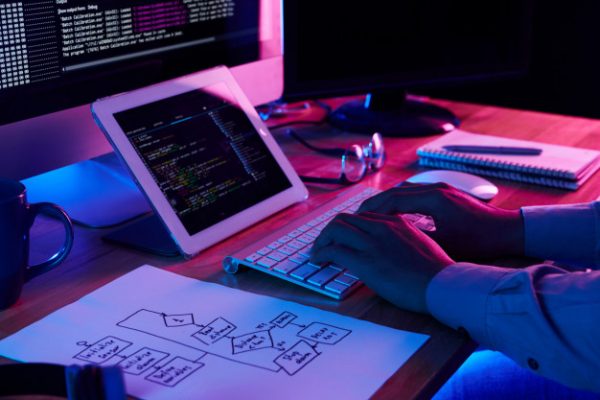
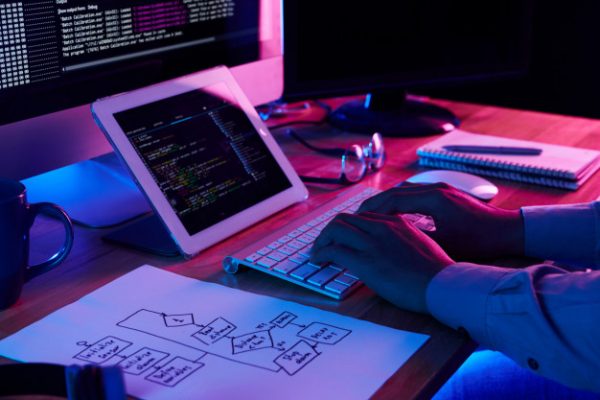
Procedural, Object-Oriented and Parallel Processing – Its participation to Imperative Programming
Imperative programming has three categories. Here are the following categories:
-
Procedural Programming
Procedural programming highlights on a procedure in terms of the machine model. This has the ability to recycle the code. Here’s a simple code snippet of how procedural programming looks like in C++ programming implementation.
#include <iostream>
using namespace std;
int main()
{
int x, y = 1, num;
cout << "Enter any Number: ";
cin >> number;
for (x = 1; x <= num; x++) {
y = y * x;
}
cout << "Factorial of " << num << " is: " << y << endl;
return 0;
}
The code above is an example of getting and displaying factorial numbers. As you can observe in the code above, it consists of procedures. These procedures are functions, routines, subroutines that carry out different computational steps. Another fact, about procedural programming, is that during execution, the procedure might be called and used at any point in the program.
-
Object-Oriented Programming(OOP)
Object-oriented programming is known for using objects and classes. Objects are defined as a data field that has unique attributes and behavior. Ultimately, objects will work together to provide functionality that your application needs. These objects are fundamental building blocks of an application which many objects maybe use in different types. These different type of object comes from a specific class of object’s type.
Classes, on the other hand, acts as the blueprint or set of instructions to create an object. Classes are designed and programmed to have one specific responsibility throughout the entire application. Here’s a simple implementation of OOP using Java program.
import java.io.*;
class Test {
public static void main(String[] args)
{
System.out.println("TEST!");
Details user_detail = new Details();
user_detail.add_account (18, "Rangie", "rangie_gwapo@gmail.com", 'M');
}
}
class Details {
int user_id;
String name;
String email_add;
char gender;
public void add_account(int user_id, String name,
String email_add, char gender)
{
System.out.println("Welcome to my page! \n Lets create your account\n");
this.user_id = 132;
this.name = "Lorly";
this.email_add = "lorly_beautiful@yahoo.com";
this.gender = 'F';
System.out.println("Account has been created");
}
}
The code above illustrates the usage of objects and classes. Its emphasis on the data rather than a procedure. Another advantage of using object-oriented programming is the flexibility and usage of abstraction that makes it easy to reuse.
-
Parallel Processing Approach
Parallel processing a.k.a. parallel computing. This approach is a method of simultaneously processing instructions and breaking up programs to multiple tasks in microprocessors. Unlike others, this can be achieved by using two or more processors. Parallel processing is practical when running programs that perform complex operations. That is why supercomputers have hundreds of microprocessors for the purpose of parallel processing. And the best programming examples of these approaches are C/C++.
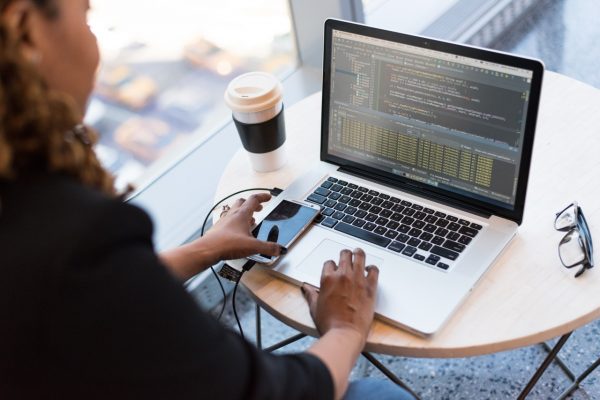
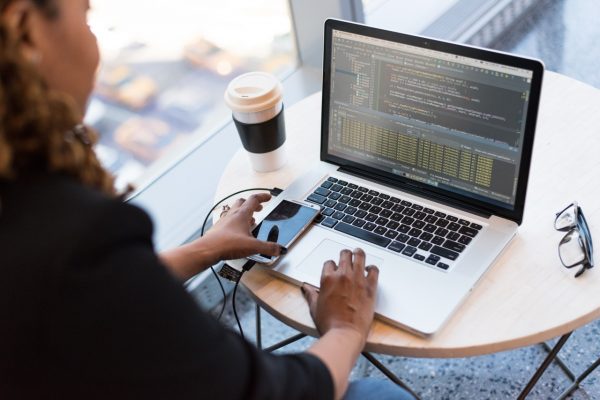
List of Imperative Programming Languages
There are a lot of programming languages in the market today. You might not be aware of some of these languages implement using an imperative programming approach. So here’s a list of programming languages that use an imperative approach.
Conclusion
Knowing the type of programming paradigm is also an advantage as a programmer or designer. This gives a guide in accomplishing some software requirements and implementation in an application. However, there’s no definite programming paradigm that fits perfectly for everyone. It all boils down to the types of applications to build, design, software requirements, and specifications.
As for this article, using imperative programming might be a start for your career in programming! For starters, it is wise to fully understand procedural programming and later on escalate to Object-oriented programming.