In non-object-oriented programming, a data structure is quite a complicated and difficult topic, especially for beginners. This is the reason we will discuss ArrayList implementation in Java in this piece. For learning and discussion purposes, this article will provide a simple sample code snippet. We will be also using Java programming language in the implementation of ArrayList. Let’s see the difference and implementation in this Java List vs ArrayList matchup in object-oriented programming.
In Java programming language, there are two ways in array implementation: fixed-sized arrays and dynamic-sized arrays. We normally first encounter normal build-in array implementation with a fixed size of an array. You cannot modify an Array size in Java but you can remove or create a new one. However, Java has found another way to solve its limitations by using ArrayList. A dynamically sized array is simply referred to as ArrayList. ArrayList is part of the java.util package and part of the collection framework—a group of objects that are consist of different classes and interface that represents as a single unit.
Both of these arrays have different syntax and structure implementation. Before proceeding to Java List vs ArrayList implementation, Let me recall to you the fixed-size array implementation.
Standard Array Implementation
In both object-oriented and non-object-oriented programming, Array is a group of variables with the same data and has a common name. Here’s a step-by-step implementation between Java List vs ArrayList in Java programming language.
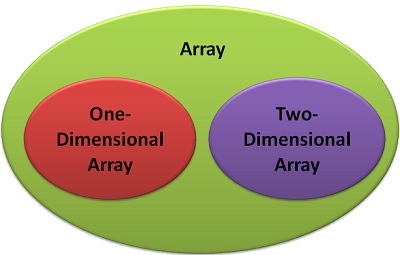
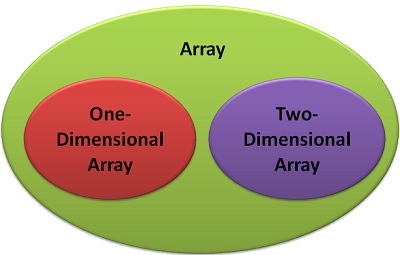
One- Dimensional Array
In Java, a one-dimensional array has a general form of either of the following:
DataType[] variable_name;
orDataType variable_name[ ];
Instantiating an array in Java programming has this general format:
Variable_name = new datatype[size];
Using the general format above, here’s an example of how a one-dimensional array is being implemented.
import java.util.*;
public class MyClass{
public static void main(String[] args){
String[] students = new String[]{“Jakeniel”, “Lorly”, “Juliet”, “Ryan”}
System.out.println(“List of Students using One Dimensional Array”);
for(int x=0; x<str.length; x++){
System.err.println(str[x] + “ “);
}
}
}
The output would then look like this:
List of Students using One Dimensional Array Jekeniel Lorly Juliet Ryan
In the example, we declared a string array with the element and print strings from the string array. It uses the for-loop structure to print the content of the String which has the index str[x]. Other implementation of a one-dimensional array does not only limit to using string but also with other data types.
import java.util.*;
public class StandardImplementation{
public static void main(String args[]){
int[] num = new int[5];
num[0] = 90;
num[1] = 30;
num[2] = 50;
num[3] = 80;
num[4] = 100;
System.out.println(“Sample code using standard implementation of One dimensional array”);
System.out.println(num[0]);
System.out.println(num[1]);
System.out.println(num[2]);
System.out.println(num[3]);
System.out.println(num[4]);
}
}
This will be the output of the code above:
Sample code using standard implementation of One dimensional array 90 30 50 80 100
The sample code is a standard array implementation using int data type. You should specify the index of the one-dimensional array in the code. Hence, Java implementation of the one-dimensional array needs to have a single index and location are specific throughout the code.
Multidimensional Array
Java also has a multidimensional array, where arrays of arrays are holding the reference of other arrays. A multidimensional array is also called Jagged Arrays, where it appends one set of square brackets per dimension. The general format for Multidimensional Array is as follows:
DataType[ ][ ] Variable_name = new DataType[size][size]; // 2D array
DataType[ ][ ][ ] Variable_name = new DataType[size][size][size]; //3D array
Let’s try to implement a multidimensional array so we can differentiate it from a one-dimensional array and dynamic ArrayList implementation. Here’s how to implement the general format given above.
import java.util.*;
public class StandardImplementation{
public static void main(String args[]){
String[][] title = {
{“Engr. ”, “Dr. ”,”Atty. ”},
{“Juliet”,”Jakeniel”}
};
System.out.println(title[0][0] + title[1][0]);
System.out.println(title[0][1] + title[1][1]);
}
}
The following would be the output of the abovementioned code:
Engr. Juliet Dr. Jakeniel
In the example, the array variable title is a 2D array where the first row has three elements and two elements are on the second raw. By using indexes, elements of a two-dimensional array can be accessed as you can see in the sample code.
ArrayList Implementation
ArrayList implements Java’s List Interface and is a part of Java’s Collection under Java Util Package. Here’s the hierarchy of ArrayList:
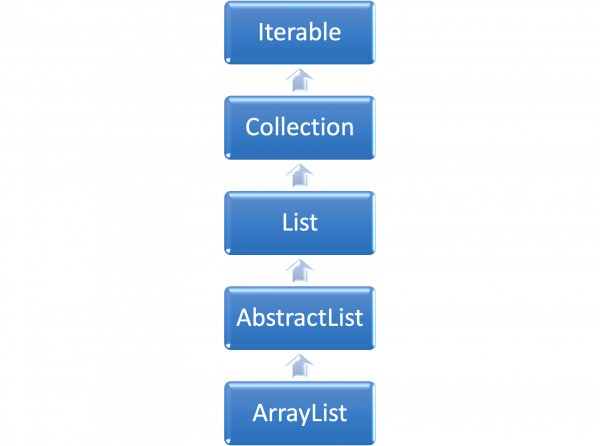
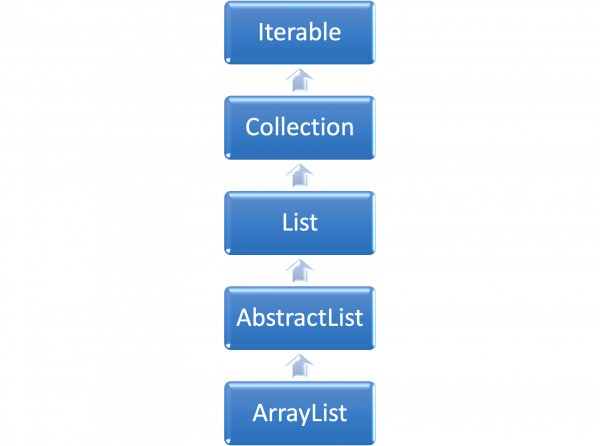
In Java programming, inheritance is a technique where one object acquires all the properties and behaviors of the parent object. Inheritance is one of the most important techniques in object-oriented programming. In the figure above, the ArrayList class extends the AbstractList class which implements List interface which extends Collection class and Iterable interface.
General Form
ArrayList implementation is slightly different from one-dimensional and multidimensional arrays in Java. Let’s take a closer look at the general format of ArrayList in the code below.
import java.util.ArrayList;
ArrayList variable_name = new ArrayList( );
In the first line of code, it imports the ArrayList class from Java packages. The second line is the general format for creating an ArrayList. Here’s a sample another example of how the general format you can use.
import java.util.ArrayList;
public class MyClass{
public static void main(String[] args){
ArrayList students = new ArrayList();
students.add(“Jakeniel”);
students.add(“Lorly”);
students.add(“Princess”);
students.add(“Melin”);
students.add(“Juliet”);
students.add(“Ryan”);
students.add(“Shirley”);
System.out.println(students);
}
}
The following will be the output:
[Jakeniel, Lorly, Princess, Melin, Juliet, Ryan, Shirley]
Using the ArrayList has different methods. In the example above, I created a class named MyClass which consists of names of students. Using variable_name.add();
is another method in the ArrayList class. This method simply adds elements to the ArrayList. Aside from the addition method, there are also other several methods in ArrayList that are useful. These methods are the following:
variable_name.set(); //refer to the index number of an element to modify
variable_name.remove(); // refer to the index number of an element to remove
variable_name.get(); // refer to the index number of an element to access
variable_name.size(); // to identify how many elements in the list
variable_name.clear(); // to remove all elements in the ArrayList
Sorting arrays in non-object oriented programming are quite a long process. With the aid of relevant classes in Java, sorting is quite easier to implement. Using the Collection class in Java, the sorting method is already incorporated and easy to implement. Here’s a sample code implementation of sorting using ArrayList in Java:
import java.util.ArrayList;
import java.util.Collections;
public class MyClass{
public static void main(String[] args){
ArrayList students = new ArrayList();
students.add(“Jakeniel”);
students.add(“Lorly”);
students.add(“Princess”);
students.add(“Melin”);
students.add(“Juliet”);
students.add(“Ryan”);
students.add(“Shirley”);
Collections.sort(students);
for(String x: students)
System.out.println(x);
}
}
The output will look like this:
[Jakeniel, Juliet, Lorly, Melin, Priincess, Ryan, Shirley]
In this code, I use Java Collection class under the java.util package. You can sort the name of students alphabetically by adding Collections.sort(variable_name)
to the code above. The output was slightly different from the previous example. Student’s names are alphabetically arranged.
Another notable usage of ArrayList using contains()
method, in which it uses a search algorithm where the specified element exists in the list given. Here’s a sample code using ArrayList contains():
import java.util.ArrayList;
public class MyClass{
public static void main(String[] args){
ArrayList students = new ArrayList();
students.add(“Jakeniel”);
students.add(“Lorly”);
students.add(“Princess”);
students.add(“Melin”);
students.add(“Juliet”);
students.add(“Ryan”);
students.add(“Shirley”);
boolean student_names = students.contains(“Juliet”);
if(student_names)
System.out.println(“The students list has name that contains Juliet”);
else
System.out.println(“No name Juliet in the list of Students”);
}
}
The result of the code will be the folowing:
The students list has name that contains Juliet
The code above uses a method in ArrayList contains()
. Use the add()
method to initialize values for student names. To check each element in the ArrayList if the student name “Juliet” exists, we can useboolean student_names = students.contains(“Juliet”)
in the code. Of course, checking will be complete all throughout with the use of a conditional statement.
Comparison of Array and ArrayList
You can use Array and ArrayList to store a group of objects, and both structures are the most useful and common data types used by most programmers. So here’s a little insight into the advantages and drawbacks of ArrayList and Array.
- The arrays do have a fixed size while ArrayList is dynamic. You cannot change the fixed-sized arrays once created.
- In an array, you cannot insert an extra element into it once you create the element. ArrayList is flexible in that matter. You can insert ArrayList or delete it in any particular position. Both Array and ArrayList can hold duplicates and multiple null elements.
- In an array, the memory is only allocated during its creation. The ArrayList has lots of methods that you can use in order to store, modify, delete and clear objects.
In the Java programming language, both Array and ArrayList provide similar performances in adding and getting an element of the indexes. For most Java programmers, the familiarity of the usage of both Array and ArrayList sometimes makes one set better than the other. At the end of the day, how you use either method will improve your programming speed.